disciplines module¶
The disciplines of the Sobieski’s SSBJ use case.
- class gemseo.problems.sobieski.disciplines.SobieskiAerodynamics(dtype='float64')[source]
Bases:
SobieskiDiscipline
Aerodynamics discipline for the Sobieski’s SSBJ use case.
Initialize self. See help(type(self)) for accurate signature.
- Parameters:
dtype (str) –
The data type for the NumPy arrays, either “float64” or “complex128”.
By default it is set to “float64”.
- classmethod create_with_physical_naming(dtype='float64')[source]
- Parameters:
dtype (str) –
The data type for the NumPy arrays, either “float64” or “complex128”.
By default it is set to “float64”.
- Return type:
- cache: AbstractCache | None
The cache containing one or several executions of the discipline according to the cache policy.
- data_processor: DataProcessor
A tool to pre- and post-process discipline data.
- dtype: str
The data type for the NumPy arrays.
- exec_for_lin: bool
Whether the last execution was due to a linearization.
- input_grammar: BaseGrammar
The input grammar.
- jac: dict[str, dict[str, ndarray]]
The Jacobians of the outputs wrt inputs.
The structure is
{output: {input: matrix}}
.
- name: str
The name of the discipline.
- output_grammar: BaseGrammar
The output grammar.
- re_exec_policy: ReExecutionPolicy
The policy to re-execute the same discipline.
- residual_variables: Mapping[str, str]
The output variables mapping to their inputs, to be considered as residuals; they shall be equal to zero.
- run_solves_residuals: bool
Whether the run method shall solve the residuals.
- sobieski_problem: SobieskiProblem
The Sobieski’s SSBJ use case defining the MDO problem, e.g. disciplines, constraints, design space and reference optimum.
- class gemseo.problems.sobieski.disciplines.SobieskiDiscipline(dtype='float64')[source]
Bases:
MDODiscipline
Abstract base discipline for the Sobieski’s SSBJ use case.
Initialize self. See help(type(self)) for accurate signature.
- Parameters:
dtype (str) –
The data type for the NumPy arrays, either “float64” or “complex128”.
By default it is set to “float64”.
- classmethod create_with_physical_naming(dtype='float64')[source]
- Parameters:
dtype (str) –
The data type for the NumPy arrays, either “float64” or “complex128”.
By default it is set to “float64”.
- Return type:
- GRAMMAR_DIRECTORY: ClassVar[str | None] = PosixPath('/home/docs/checkouts/readthedocs.org/user_builds/gemseo/envs/5.0.0/lib/python3.9/site-packages/gemseo/problems/sobieski/grammars')
The directory in which to search for the grammar files if not the class one.
- cache: AbstractCache | None
The cache containing one or several executions of the discipline according to the cache policy.
- data_processor: DataProcessor
A tool to pre- and post-process discipline data.
- dtype: str
The data type for the NumPy arrays.
- exec_for_lin: bool
Whether the last execution was due to a linearization.
- input_grammar: BaseGrammar
The input grammar.
- jac: dict[str, dict[str, ndarray]]
The Jacobians of the outputs wrt inputs.
The structure is
{output: {input: matrix}}
.
- name: str
The name of the discipline.
- output_grammar: BaseGrammar
The output grammar.
- re_exec_policy: ReExecutionPolicy
The policy to re-execute the same discipline.
- residual_variables: Mapping[str, str]
The output variables mapping to their inputs, to be considered as residuals; they shall be equal to zero.
- run_solves_residuals: bool
Whether the run method shall solve the residuals.
- sobieski_problem: SobieskiProblem
The Sobieski’s SSBJ use case defining the MDO problem, e.g. disciplines, constraints, design space and reference optimum.
- class gemseo.problems.sobieski.disciplines.SobieskiMission(dtype='float64', enable_delay=False)[source]
Bases:
SobieskiDiscipline
Mission discipline of the Sobieski’s SSBJ use case.
Compute the range with the Breguet formula.
Initialize self. See help(type(self)) for accurate signature.
- Parameters:
dtype (str) –
The data type for the NumPy arrays, either “float64” or “complex128”.
By default it is set to “float64”.
If
True
, wait one second before computation. If a positive number, wait the corresponding number of seconds. IfFalse
, compute directly.By default it is set to False.
- classmethod create_with_physical_naming(dtype='float64', enable_delay=False)[source]
- Parameters:
- Return type:
- cache: AbstractCache | None
The cache containing one or several executions of the discipline according to the cache policy.
- data_processor: DataProcessor
A tool to pre- and post-process discipline data.
- dtype: str
The data type for the NumPy arrays.
- enable_delay: bool | float
If
True
, wait one second before computation.If a positive number, wait the corresponding number of seconds. If
False
, compute directly.
- exec_for_lin: bool
Whether the last execution was due to a linearization.
- input_grammar: BaseGrammar
The input grammar.
- jac: dict[str, dict[str, ndarray]]
The Jacobians of the outputs wrt inputs.
The structure is
{output: {input: matrix}}
.
- name: str
The name of the discipline.
- output_grammar: BaseGrammar
The output grammar.
- re_exec_policy: ReExecutionPolicy
The policy to re-execute the same discipline.
- residual_variables: Mapping[str, str]
The output variables mapping to their inputs, to be considered as residuals; they shall be equal to zero.
- run_solves_residuals: bool
Whether the run method shall solve the residuals.
- sobieski_problem: SobieskiProblem
The Sobieski’s SSBJ use case defining the MDO problem, e.g. disciplines, constraints, design space and reference optimum.
- class gemseo.problems.sobieski.disciplines.SobieskiPropulsion(dtype='float64')[source]
Bases:
SobieskiDiscipline
Propulsion discipline of the Sobieski’s SSBJ use case.
Initialize self. See help(type(self)) for accurate signature.
- Parameters:
dtype (str) –
The data type for the NumPy arrays, either “float64” or “complex128”.
By default it is set to “float64”.
- classmethod create_with_physical_naming(dtype='float64')[source]
- Parameters:
dtype (str) –
The data type for the NumPy arrays, either “float64” or “complex128”.
By default it is set to “float64”.
- Return type:
- cache: AbstractCache | None
The cache containing one or several executions of the discipline according to the cache policy.
- data_processor: DataProcessor
A tool to pre- and post-process discipline data.
- dtype: str
The data type for the NumPy arrays.
- exec_for_lin: bool
Whether the last execution was due to a linearization.
- input_grammar: BaseGrammar
The input grammar.
- jac: dict[str, dict[str, ndarray]]
The Jacobians of the outputs wrt inputs.
The structure is
{output: {input: matrix}}
.
- name: str
The name of the discipline.
- output_grammar: BaseGrammar
The output grammar.
- re_exec_policy: ReExecutionPolicy
The policy to re-execute the same discipline.
- residual_variables: Mapping[str, str]
The output variables mapping to their inputs, to be considered as residuals; they shall be equal to zero.
- run_solves_residuals: bool
Whether the run method shall solve the residuals.
- sobieski_problem: SobieskiProblem
The Sobieski’s SSBJ use case defining the MDO problem, e.g. disciplines, constraints, design space and reference optimum.
- class gemseo.problems.sobieski.disciplines.SobieskiStructure(dtype='float64')[source]
Bases:
SobieskiDiscipline
Structure discipline of the Sobieski’s SSBJ use case.
Initialize self. See help(type(self)) for accurate signature.
- Parameters:
dtype (str) –
The data type for the NumPy arrays, either “float64” or “complex128”.
By default it is set to “float64”.
- classmethod create_with_physical_naming(dtype='float64')[source]
- Parameters:
dtype (str) –
The data type for the NumPy arrays, either “float64” or “complex128”.
By default it is set to “float64”.
- Return type:
- cache: AbstractCache | None
The cache containing one or several executions of the discipline according to the cache policy.
- data_processor: DataProcessor
A tool to pre- and post-process discipline data.
- dtype: str
The data type for the NumPy arrays.
- exec_for_lin: bool
Whether the last execution was due to a linearization.
- input_grammar: BaseGrammar
The input grammar.
- jac: dict[str, dict[str, ndarray]]
The Jacobians of the outputs wrt inputs.
The structure is
{output: {input: matrix}}
.
- name: str
The name of the discipline.
- output_grammar: BaseGrammar
The output grammar.
- re_exec_policy: ReExecutionPolicy
The policy to re-execute the same discipline.
- residual_variables: Mapping[str, str]
The output variables mapping to their inputs, to be considered as residuals; they shall be equal to zero.
- run_solves_residuals: bool
Whether the run method shall solve the residuals.
- sobieski_problem: SobieskiProblem
The Sobieski’s SSBJ use case defining the MDO problem, e.g. disciplines, constraints, design space and reference optimum.
- gemseo.problems.sobieski.disciplines.create_disciplines(dtype='float64')[source]
Instantiate the structure, aerodynamics, propulsion and mission disciplines.
- Parameters:
dtype (str) –
The NumPy type for data arrays, either “float64” or “complex128”.
By default it is set to “float64”.
- Returns:
The structure, aerodynamics, propulsion and mission disciplines.
- Return type:
list[gemseo.problems.sobieski.disciplines.SobieskiDiscipline]
- gemseo.problems.sobieski.disciplines.create_disciplines_with_physical_naming(dtype='float64')[source]
Instantiate the structure, aerodynamics, propulsion and mission disciplines.
Use a physical naming for the input and output variables.
- Parameters:
dtype (str) –
The NumPy type for data arrays, either “float64” or “complex128”.
By default it is set to “float64”.
- Returns:
The structure, aerodynamics, propulsion and mission disciplines.
- Return type:
Examples using SobieskiAerodynamics¶
Examples using SobieskiMission¶
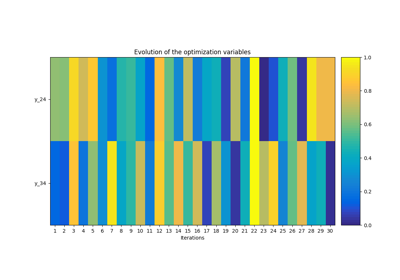
Simple disciplinary DOE example on the Sobieski SSBJ test case